In my last post on C# concepts we talked about variables and data types. In this next post on C# basics, we'll look at ways of implementing conditional logic in C#. We'll look at things like if statements, switch statements, conditional operators and more. Be sure to subscribe if you're enjoying my content on C#.
If, else if and else statements
The first one which translates from almost all languages is if statements. Let's take a look at how we implement these in C#. In this scenario I want to achieve the following logic. Let's write the pseudocode first...
START
SET temperature TO 30
IF temperature > 30 THEN
PRINT "It's a hot day."
ELSE IF temperature >= 20 AND temperature <= 30 THEN
PRINT "It's a warm day."
ELSE
PRINT "It's a cool day."
END IF
END
Pseudocode for temperature logic
Now let's see how we'd implement this, using If, else if, and else in C#
int temperature = 30;
if (temperature > 30)
{
Console.WriteLine("It's a hot day.");
}
else if (temperature >= 20 && temperature <= 30)
{
Console.WriteLine("It's a warm day.");
}
else
{
Console.WriteLine("It's a cool day.");
}
Pseudocode for temperature logic
Here we see how logic we wanted to implement translates into an if statement in C#. We start with the if keyword then we assess an expression within rounded brackets before opening curly braces to execute statements. Then for any other conditions that we want to add that don't pass the first condition, we add an else if block, and we finish with an else block for any values returned which don't match any of the expressions assessed so far.
Switch statement
Now let's look at switch statements, which are incredibly similar to if statements but tend to be a lot more helpful when we have lots of expressions and scenarios to assess against a single value which could be a variable or expression.
Let's look at a switch statement in C# now...
switch (expression)
{
case value1:
// Code to execute if expression equals value1
break;
case value2:
// Code to execute if expression equals value2
break;
// Add more cases as needed
default:
// Code to execute if expression does not match any case
break;
}
Commented C# to demonstrate a switch statement.
Here in the statement you can see we start with the switch keyword. Then in the rounded brackets we provide the expression to assess. This is then the value which we will assess switch cases against. Next, within the curly braces we add each case using the case keyword followed by the value to compare then a colon which notes the next lines being statements to execute if that case passes. Then each case is closed with the break keyword and a semi-colon. At the end of a switch statement still within the curly braces, we add the default keyword followed by a colon and execution statements for a fallback in case the expression passed into the statement doesn't match any specific case. Then we finish with break; again.
Switch expression
A simplified version of a switch statement which we can use is the switch expression. This is something which was introduced to C# in version 8.0 and follows a syntax like below:
int dayOfWeek = 5;
string dayName = dayOfWeek switch
{
1 => "Monday",
2 => "Tuesday",
3 => "Wednesday",
4 => "Thursday",
5 => "Friday",
6 => "Saturday",
7 => "Sunday",
_ => "Um... yup, not a day of the week *insert side eye emoji*"
};
Console.WriteLine(dayName);
Pseudocode for days of the week switch statement
In this syntax, we create a new variable to assign the result of the switch expression to. Then after the equals, we provide the expression or the value to assess against the switch cases. Then we use the switch keyword, open curly braces and use the following syntax: case/matching value => result value,. Then in place of the default keyword we use an underscore _ followed by the same syntax => result value then followed by a closing curly brace and a semi colon.
This allows us to write more concise code in C#. Writing a full switch statement is potentially more comparable to plain english and may be easier to read if there aren't countless cases.
Conditional operator
The conditional operator is one of my favourite methods of implementing simple conditional logic in C# and is a simplified alternative to writing a full if statement. I suppose it is similar to a switch expression when compared to a switch statement. There is perhaps the consideration that both are written in less plain english and are less easy to understand for a very early beginner to C#. This isn't too difficult to understand though, let's walk through it...
This is the syntax for the conditional operator...
condition ? first_expression : second_expression;
Conditional operator syntax
Here the condition is the expression that we will evaluate or the value we evaluate. Then we use a ? followed by the expression to evaluate and return if the first expression returns a true value, the then following the colon (:) we provide the value returned if the condition is false.
Keep in mind that the condition should return a bool value (true / false).
What if it's a null?
To finish up, whilst we could continue to go on with other syntax's, let's look at how we can handle potential null values with the null-coalescing operator and assignment operator.
?? Null-Coalescing Operator
First up we have the null-coalescing operator which we can use to provide a fallback value to handle a null value as a result to an expression. Let's look at an example...
string blogName = null;
string siteName = blogName ?? "LewisDoesDev";
Console.WriteLine(siteName);
Null-coalescing operator in C#
In the above example which demonstrates the null-coalescing operator ??
. We can see the we start by creating a variable and assigning a null value so we can later use that as an expression which will return a null value. Then we create a new variable and assign the blogName variable as it's value. In this scenario we know the value will be null. But in a scenario where we don't ever want a null value, we can follow up the expression with ??
followed by a fallback hard coded value or another expression. This results in us being able to create fallbacks for expressions which result in a null value.
??= Null-Coalescing Assignment Operator
The other thing we have is the equivalent assignment operator which assigns a fallback value to the left-hadn operand if the left-hand operand is null. Let's take the previous code and shape it to this example...
string blogName = null;
blogName ??= "LewisDoesDev";
Console.WriteLine(blogName);
Null-coalescing operator in C#
In this example, you can see that the assignment operator assesses whether the variable blogName is equal to a null value, and if it is, it then conditionally (true) assigns the right-hand operand value, in this case "LewisDoesDev".
Time to recap
There are several additional examples of ways to implement conditional logic which we could talk about, but for now, let's recap and test your knowledge before going further with Microsoft Learn.
How can we implement simple conditional logic for a few cases assessed against an expression?
We can use an if, else if, else statement for branching or implementing conditional logic where there are a few different cases and comparisons to assess.
What should we use if we're assessing several different cases against a single expression?
A switch statement or expression sounds like the match for this scenario! A switch statement is best for when we 1. are assessing cases against one consistent value / expression, and 2. when we have a higher number of cases to assess.
Is there a condensed alternative to writing full if statements?
Sounds like we can use the conditional operator syntax for this. condition ? true value : false value
How do we handle null values in C#?
In this scenario, to prevent null values in our logic, we can use the null-coalescing operator and assignment operator to apply a fallback to null values that are evaluated.
Continue learning at Microsoft Learn...
Want to take your learning further? Here are the Microsoft Learn pathways and modules I think might be best for you to pick up on next...
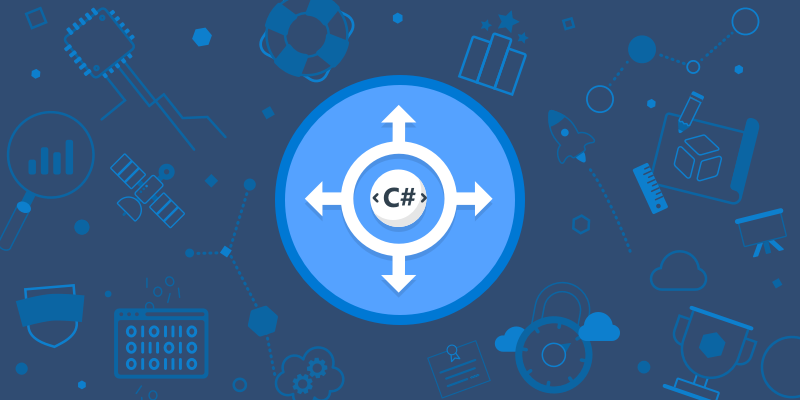
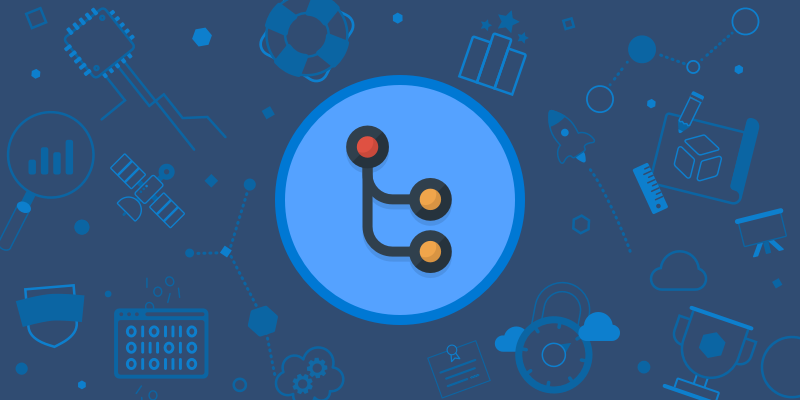
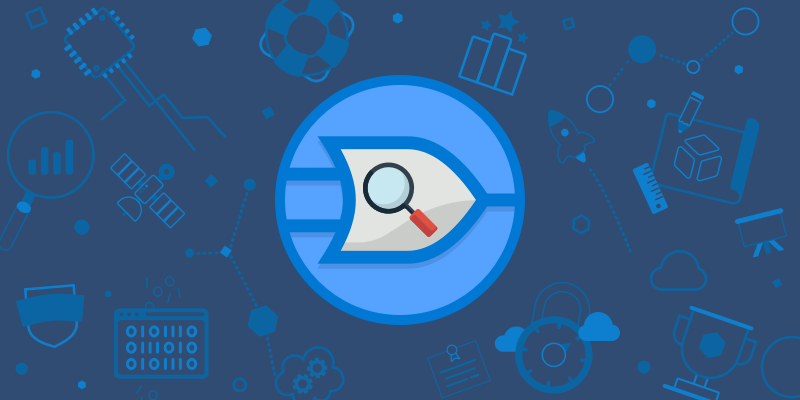
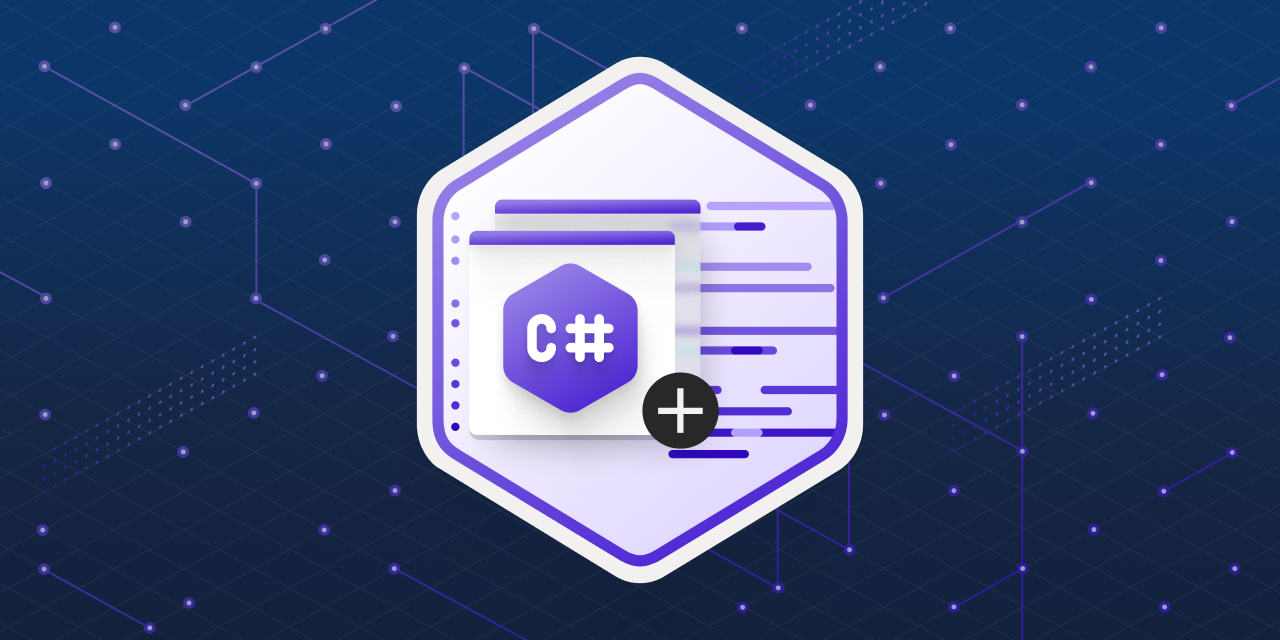
Welcome to LewisDoesDev π
If you're new here, welcome to LewisDoesDev. I'm Lewis, and whilst C# is one topic I write about I also blog about topics surrounding...
- Microsoft 365
- Power Platform
- Dynamics 365
- Microsoft Graph
- Azure
- dotnet
- Copilot and more!
If that sounds interesting to you, be sure to subscribe to my free content today so you don't miss a post!